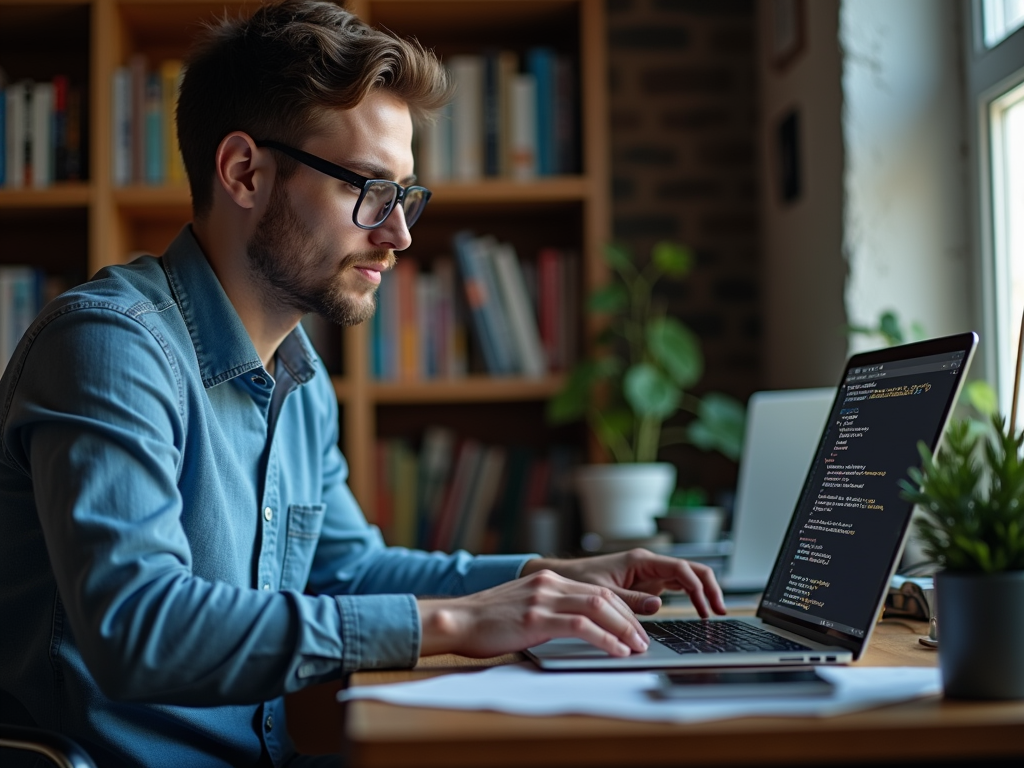
In the world of JavaScript programming, the concept of a dictionary serves as a vital tool for organizing and managing data. A JavaScript dictionary allows developers to store, retrieve, and manipulate data in a format that is easily accessible. What makes these dictionaries so powerful is their ability to function as dynamic entities, adapting to the needs of the program as it evolves. With multiple methods for creation and manipulation, it’s essential to grasp the various approaches available. Understanding this allows you to choose the method that best fits your project requirements while enhancing code readability and efficiency. Let’s delve deeper into the fascinating world of JavaScript dictionaries, where simplicity meets versatility.
JavaScript dictionaries can be broadly categorized into two types: objects and maps. While both serve equivalent purposes, the nuances in their structures lead to distinctive performance attributes and uses scenarios. As we explore these differences, you will find that leveraging the right type of dictionary will significantly enhance your development experience. This article dissects how to create, manipulate, and optimize your JavaScript dictionaries. Through clear examples and practical advice, we aim to equip you with the knowledge needed to harness the full potential of these data structures.
Understanding the Basics of JavaScript Objects
JavaScript objects are fundamental constructs that embody key-value pairs, laying the groundwork for what we refer to as dictionaries. They can hold any data type, making them extremely flexible for various use cases. Let’s take a moment to understand their properties and methods that define their utility:
- Key-Value Pairs: Objects can hold multiple data entries, where you can define and access properties using a unique key.
- Built-in Methods: Objects come with numerous built-in methods such as Object.keys(), Object.values(), and Object.entries(), making data manipulation straightforward.
- Prototype Chain: Understanding the prototype chain is crucial, as it allows inheritance and enhances object functionality.
These characteristics allow developers to employ objects as dictionaries seamlessly, making the language even more versatile. The simplicity of using objects for data storage makes them a preferred choice for many developers, especially when working on smaller projects. As the demands of JavaScript programming continue to grow, mastering these elements is paramount. In addition to their inherent properties, knowing how to manipulate JavaScript objects enhances your overall coding prowess.
Creating a Simple JavaScript Dictionary
The beauty of JavaScript lies in its ability to create dictionaries effortlessly. You can utilize different methods, each offering its own advantages. For instance, let’s explore how to create a basic dictionary using object literal notation:
Using Object Literal Notation
Object literal notation is arguably the most common way to create a JavaScript dictionary. The syntax is straightforward and looks like this:
Key | Value |
---|---|
name | John Doe |
age | 30 |
occupation | Developer |
As illustrated above, an object can be defined with multiple key-value pairs. This is the foundation upon which more complex data management can be built.
Using the `new Object()` Constructor
Another approach involves the use of the new Object() constructor. This method is particularly useful if you prefer a more structured way of creating objects. Below is a sample code snippet:
- let person = new Object();
- person.name = “Jane Doe”;
- person.age = 28;
Through either method, creating a basic dictionary in JavaScript is not only simple but also provides immense flexibility in data management. Deciding between these two methods often comes down to personal preference and specific use cases.
Advanced JavaScript Dictionaries: Using Maps
While JavaScript objects are the traditional approach, the Map object is becoming increasingly popular among developers. Maps introduce various features that enhance usability. One of the main attractions is their capacity to hold keys of any data type, providing greater flexibility.
Choosing between objects and maps can depend on several factors. Here are some key benefits of using maps:
- Key Types: Maps can have keys of any type, allowing for a greater variety of data management.
- Ordering: Maps maintain the insertion order of entries, enabling you to preserve the sequence in which data is added.
- Performance Optimization: Maps can offer better performance when it comes to frequent additions and removals of key-value pairs.
By leveraging the capabilities of maps, developers can create more efficient applications that meet the complex demands of modern software. Understanding when to use each structure will elevate your coding skills and significantly affect your project’s performance.
Conclusion
In short, understanding how to create and manipulate dictionaries in JavaScript is fundamental for efficient programming. Whether you decide on standard objects or the modern Map, each method has unique benefits that cater to different project needs. The ability to organize data flexibly and effectively is a game-changer in your coding journey. By applying the techniques and best practices discussed, you gain a solid foothold in efficient data management and code clarity.
Frequently Asked Questions
- What is the difference between an object and a Map in JavaScript? Objects are traditional key-value pairs, while Maps provide more flexibility in key types and maintain the order of elements.
- Can objects have properties with non-string keys? No, in objects, keys are automatically converted to strings. However, Maps can have keys of any type.
- How do I loop through a JavaScript object? You can use for…in, or the Object.keys(), Object.values(), or Object.entries() methods to iterate over properties.
- Are Maps more performant than objects? Maps are generally more efficient for scenarios involving frequent additions and removals of key-value pairs, primarily due to their optimized structure.